|
为了在使用 .NET 核心框架进行开发时生成 OAS 文件,开发人员可以使用:
- 虚张声势 (https://docs.microsoft.com/en-us/aspnet/core/tutorials/getting-started-with-swashbuckle?view=aspnetcore-5.0&tabs=visual-studio-code)
- 新南威尔士州 – https://docs.microsoft.com/en-us/aspnet/core/tutorials/getting-started-with-nswag?view=aspnetcore-5.0&tabs=visual-studio
使用虚张声势
在本文档的示例中,我们有一个实体(Person),它将表示 JSON 架构,以及一个控制器(人员控制器),它将为我们公开 API 的操作。
Swagger-UI 看起来非常类似于下图:
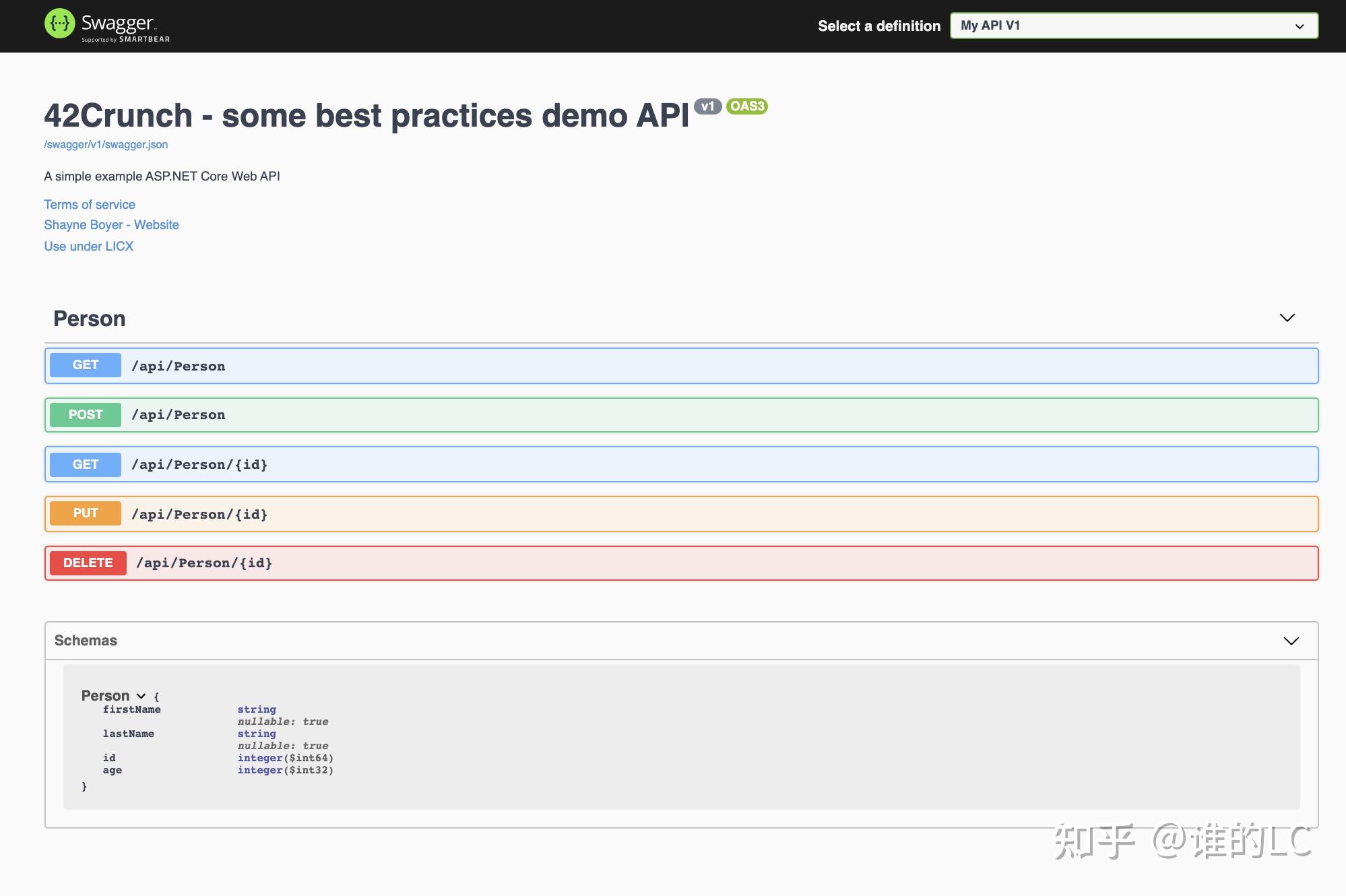
图 1 – 显示 API 操作和架构的 Swagger UI
42压缩 IDE 支持
基于 .net 框架在 Swashbuckle 支持下生成的开放 API 规范文件,我们可以获取此文件并将其添加到我们的 IDE 中,只是为了开始执行安全审核分数的过程,以确保我们的 API 中存在潜在漏洞,请参考以下使用 Visual Studio 代码和 42Crunch 扩展名的屏幕截图:
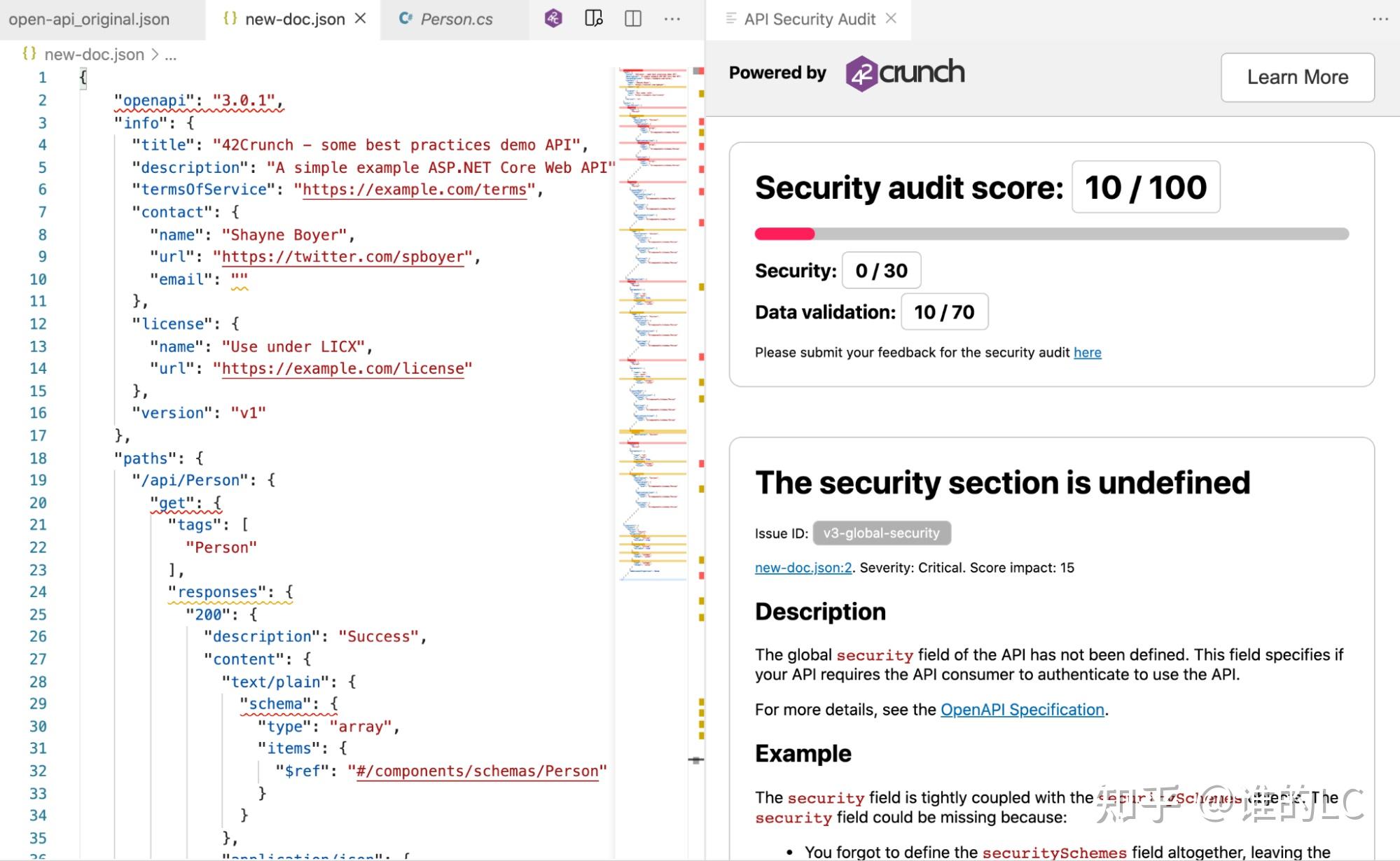
图2 – 由虚张声势生成的初始分数
42Crunch 的 Visual Studio 代码扩展为我们提供了以下审核分数:10(共 100 分),在本文档的其余部分,我们将介绍如何提高此分数,从而从根本上改进负责该分数的 C# 代码。
来自42的推荐
42Crunch的大多数建议都可以直接从代码中修复,以保护您的API免受首选IDE或通过CI / CD过程。我们将始终仅查看生成的OAS / Swagger文件,以确定您的API是否存在潜在的安全漏洞。
注意:请记住,即使拥有最好的代码和后端组件的整个覆盖范围,也不一定意味着您的API免受潜在攻击。
让我们先来看看 Person 实体,也就是我们的架构:
实体/JSON 架构的原始代码
using System.ComponentModel.DataAnnotations;
namespace TodoApi.Models {
public class Person
{
public string firstName { get; set; }
public string lastName { get; set; }
public long id { get; set; }
public int age { get; set;
} } }实体/JSON 架构的增强代码
using System.ComponentModel.DataAnnotations;
using System;
namespace TodoApi.Models {
public class Person
{ [Required, RegularExpression("/^[a-zA-Z ]{2,100}$/"),MinLength(5), MaxLength(100)]
public string firstName { get; set; }
[Required, RegularExpression("/^[a-zA-Z ]{2,100}$/"),MinLength(5), MaxLength(100)]
public string lastName { get; set; } [Required, Range(1, long.MaxValue)]
public long id { get; set; } [Required, Range(0, 150)]
public int age { get; set;
} } }让我们看看这个微小的变化如何影响了我们新的审计分数:
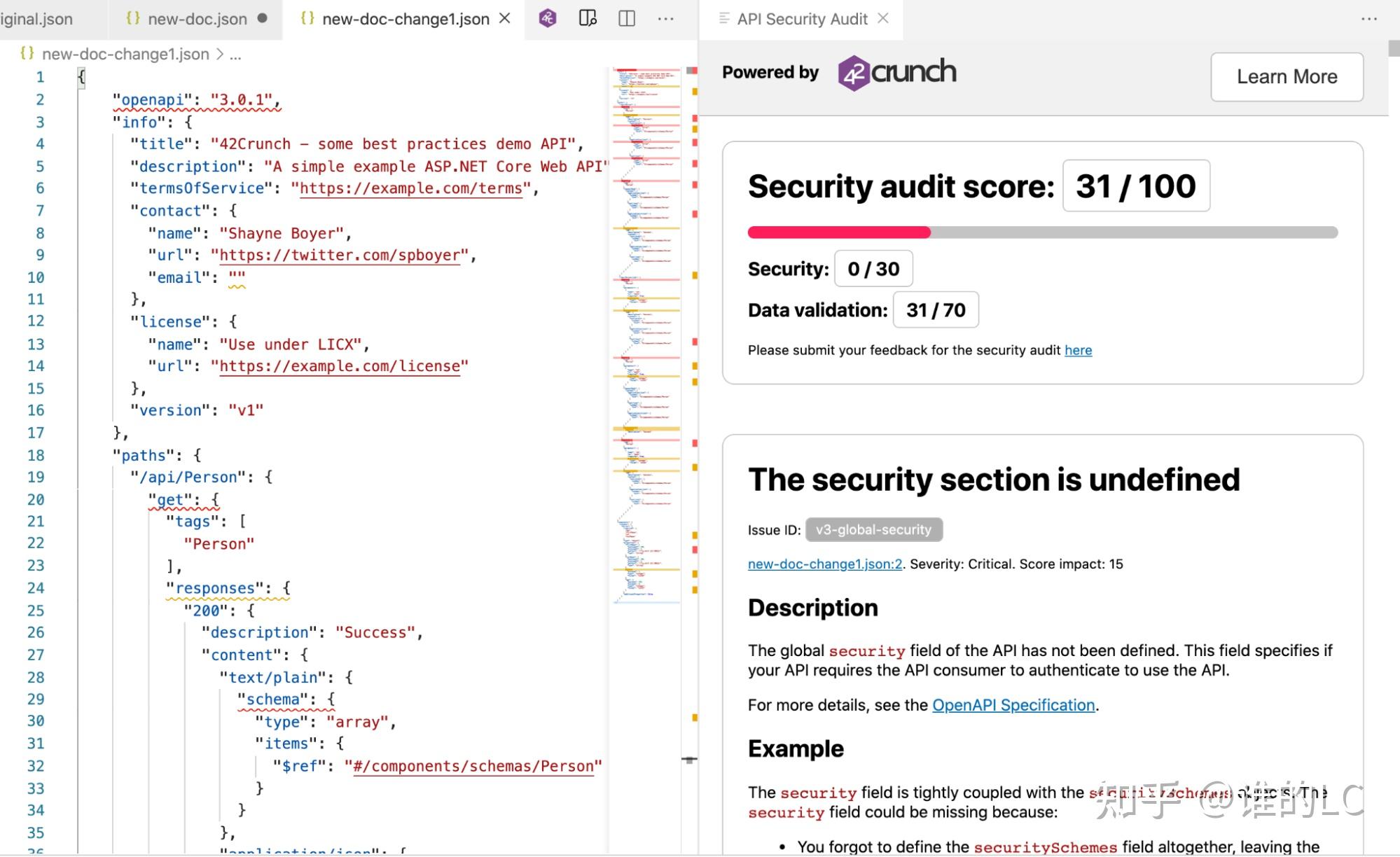
图3 – 只需稍作改动,我们的审计分数就大大提高
最关键的方面之一是未定义的安全性,因此我们将展示如何将其添加到代码广告中,如下所示:
private static void addSec(IServiceCollection services)
{
//Swaggers with Security
services.AddSwaggerGen(c =>
{
// configure SwaggerDoc and others
c.SwaggerDoc("v1", new OpenApiInfo
{
Version = "v1",
Title = "42Crunch - some best practices demo API",
Description = "A simple example ASP.NET Core Web API",
TermsOfService = new Uri("Example Domain"),
Contact = new OpenApiContact
{
Name = "Shayne Boyer",
Email = string.Empty,
Url = new Uri("https://twitter.com/spboyer"),
},
License = new OpenApiLicense
{
Name = "Use under LICX",
Url = new Uri("Example Domain"),
}
});
// jwt
// add JWT Authentication
var securityScheme = new OpenApiSecurityScheme
{
Name = "JWT Authentication",
Description = "Enter JWT Bearer token **_only_**",
In = ParameterLocation.Header,
Type = SecuritySchemeType.Http,
Scheme = "bearer", // must be lower case
BearerFormat = "JWT",
Reference = new OpenApiReference
{
Id = JwtBearerDefaults.AuthenticationScheme,
Type = ReferenceType.SecurityScheme
}
};
c.AddSecurityDefinition(securityScheme.Reference.Id, securityScheme);
c.AddSecurityRequirement(new OpenApiSecurityRequirement
{
{securityScheme, new string[] { }}
});
// add Basic Authentication
var basicSecurityScheme = new OpenApiSecurityScheme
{
Type = SecuritySchemeType.Http,
Scheme = "basic",
Reference = new OpenApiReference { Id = "BasicAuth", Type = ReferenceType.SecurityScheme }
};
c.AddSecurityDefinition(basicSecurityScheme.Reference.Id, basicSecurityScheme);
c.AddSecurityRequirement(new OpenApiSecurityRequirement
{
{basicSecurityScheme, new string[] { }}
});
});
}现在,是时候改进 API 的操作了,为此,对于 .net,我们必须研究一下控制器组件。请参阅原始人员控制器:
人员控制器的原始代码.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using Microsoft.EntityFrameworkCore;
using TodoApi.Models;
namespace TodoApi.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class PersonController : ControllerBase
{
private readonly PersonContext _context;
public PersonController(PersonContext context)
{
_context = context;
}
// GET: api/Person
[HttpGet]
public async Task<ActionResult<IEnumerable>> GetPersons()
{
return await _context.Persons.ToListAsync();
}
// GET: api/Person/5
[HttpGet(&#34;{id}&#34;)]
public async Task<ActionResult> GetPerson(long id)
{
var person = await _context.Persons.FindAsync(id);
if (person == null)
{
return NotFound();
}
return person;
}
// PUT: api/Person/5
// To protect from overposting attacks, enable the specific properties you want to bind to, for
// more details, see https://go.microsoft.com/fwlink/?linkid=2123754.
[HttpPut(&#34;{id}&#34;)]
public async Task PutPerson(long id, Person person)
{
if (id != person.id)
{
return BadRequest();
}
_context.Entry(person).State = EntityState.Modified;
try
{
await _context.SaveChangesAsync();
}
catch (DbUpdateConcurrencyException)
{
if (!PersonExists(id))
{
return NotFound();
}
else
{
throw;
}
}
return NoContent();
}
// POST: api/Person
// To protect from overposting attacks, enable the specific properties you want to bind to, for
// more details, see https://go.microsoft.com/fwlink/?linkid=2123754.
[HttpPost]
public async Task<ActionResult> PostPerson(Person person)
{
_context.Persons.Add(person);
await _context.SaveChangesAsync();
return CreatedAtAction(&#34;GetPerson&#34;, new { id = person.id }, person);
}
// DELETE: api/Person/5
[HttpDelete(&#34;{id}&#34;)]
public async Task<ActionResult> DeletePerson(long id)
{
var person = await _context.Persons.FindAsync(id);
if (person == null)
{
return NotFound();
}
_context.Persons.Remove(person);
await _context.SaveChangesAsync();
return person;
}
private bool PersonExists(long id)
{
return _context.Persons.Any(e => e.id == id);
}
}
}现在,让我们从注释中添加一些注释,以提高我们的审核分数,同时我们也在提高 API 保护级别。我们将所需的注释添加到对象中,这些注释将成为 JSON 架构生成的基础。

一些初步结果:
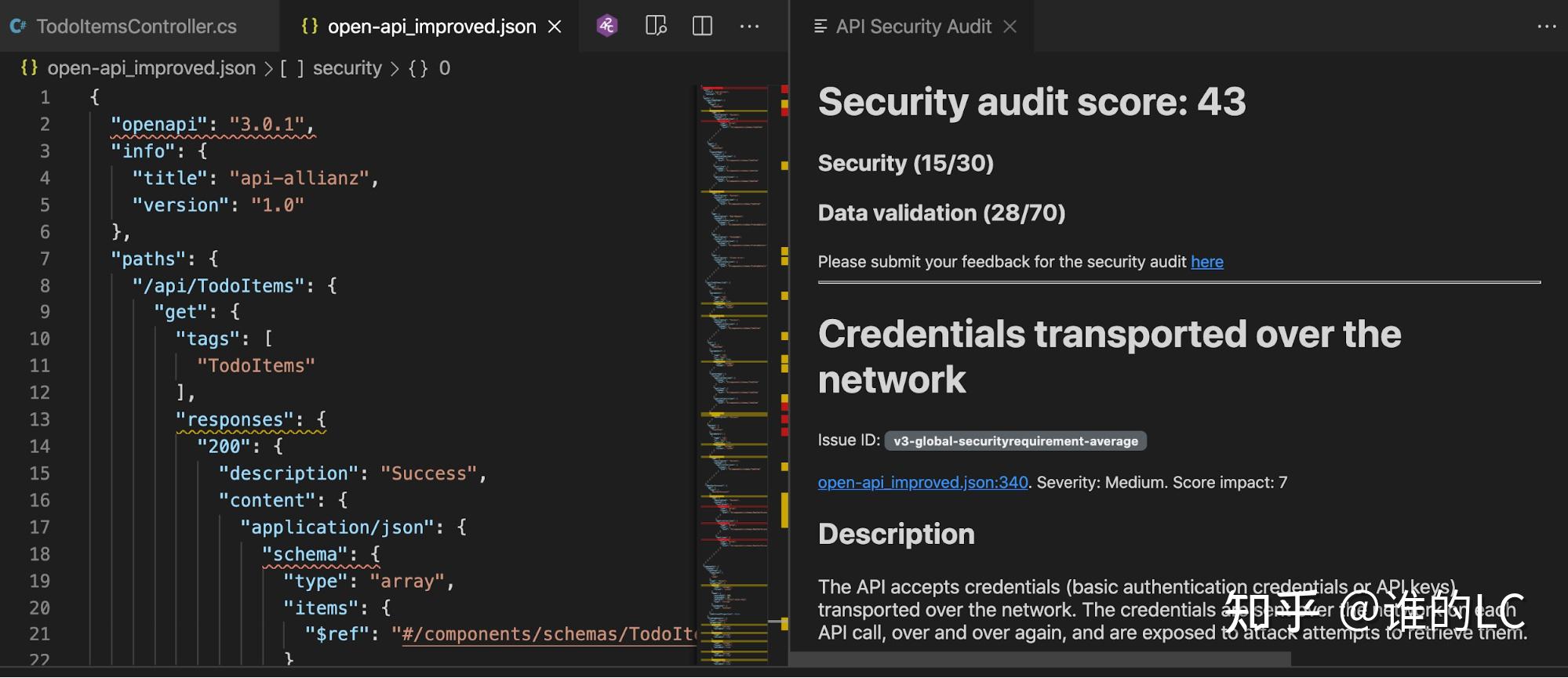
如果我们进行这些更改,我们可以将 API 的合规性状况从 16% 安全改进为 43%。这些建议不需要更改生成的 swagger,而是利用 C# Code 中的批注。
使用国家计算机
NSwag具有与“虚张声势”类似的功能。任何一个都可以配置为实现高质量的开放API规范/炫耀者。
对于管道和 CI/CD
通过 REST API 安全测试管道,您可以将我们的自动测试和验证添加到 CI/CD 管道,以便在每次 CI/CD 调用时测试 API。我们让客户使用各种工具来执行此实践,其中一些是:詹金斯、Azure 管道、比特桶、竹子等。我们还可以使用我们的API快速轻松地进行自定义集成。请在此处查看更多信息:https://42crunch.com/resources-free-tools/
一旦 API 达到可接受的审核分数(我们的建议至少为 70 分(共 100 分),它们就可以传递到 CI/CD 管道,如詹金斯、GitLab、Azure 管道、GitHub、奔步等。此外,42Crunch还提供了与许多高质量工具集成的能力,因为其中之一是声纳Qube:
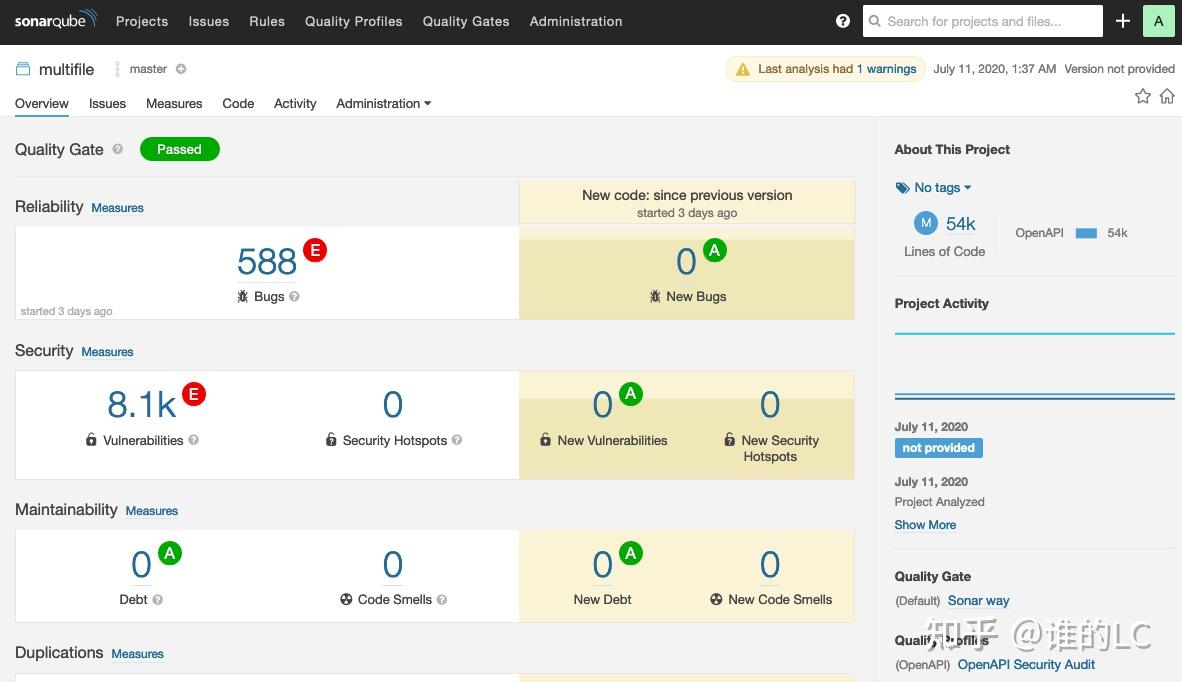
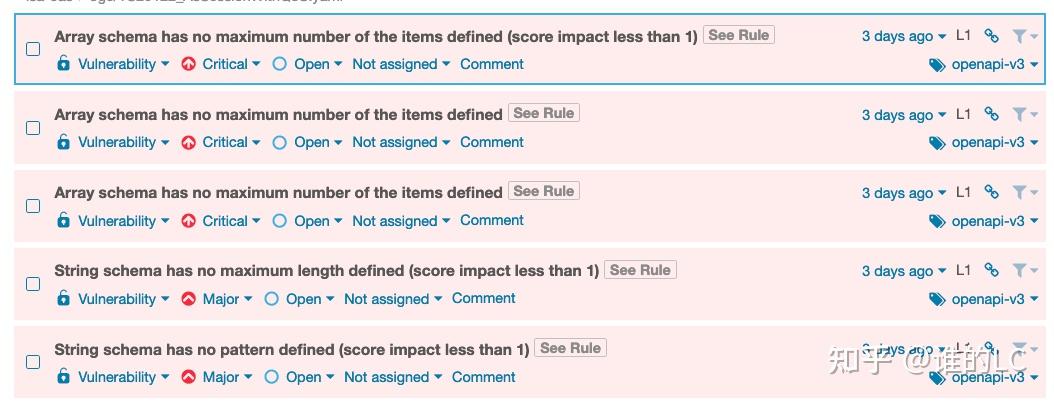
结论
42Crunch平台专注于开放API / Swagger文件,它使我们的客户能够拥有一个完全集成的保护平台,该平台了解从开始/设计到生产的API行为。依赖 Spec 文件是避免猜测什么以及如何 保护您的 API 的方法。
参考:42Crunch |
|