|
@TOC
网络编程
网络编程: 通过网络协议和双方的IP地址,实现数据交换和通信。属于C/S架构 网络模型以及对应的协议:(了解即可,计网会学)
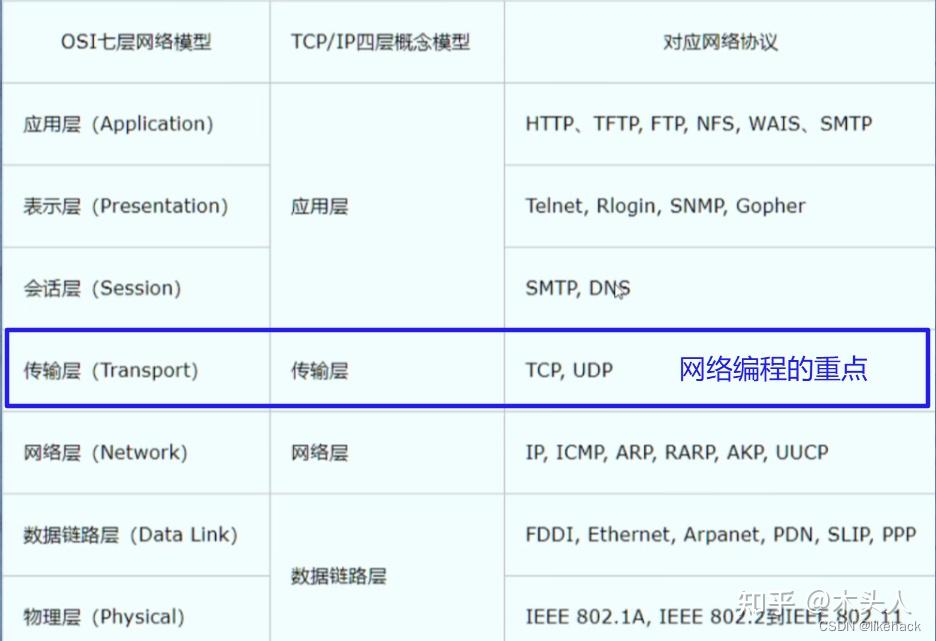
在这里插入图片描述
TCP/UDP这两个通信协议是网络编程实现通信的重点,后续会有相关案例进行讲解。
实现通信需要的条件: - IP - 端口号 - 满足网络协议
IP:网络互连协议
Java中的IP地址通过InetAddress类来实现。IP是用来定位一台网络上的计算机的唯一标识。域名是用于解决IP地址不方便记忆的问题。
IP地址的分类
- IPV4
- IPV6
- 128位,由8个无符号整数组成。如:fe80::3700:1400:3d00:cc0b%0
- 公网(互联网)
- A类地址
- 在IP地址的四段号码中,第一段号码为网络号码,剩下的三段号码为本地计算机的号码。
- A类IP地址中网络的标识长度为8位,主机标识的长度为24位,A类网络地址数量较少,有126个网络,每个网络可以容纳主机数达1600多万台。
- A类IP地址的地址范围是1.0.0.0到127.255.255.255
- B类地址
- 在IP地址的四段号码中,前两段号码为网络号码。
- B类IP地址中网络的标识长度为16位,主机标识的长度为16位,B类网络地址适用于中等规模的网络,有16384个网络,每个网络所能容纳的计算机数为6万多台。
- B类IP地址地址范围128.0.0.0-191.255.255.255
- C类地址
- 在IP地址的四段号码中,前三段号码为网络号码,剩下的一段号码为本地计算机的号码。
- C类IP地址中网络的标识长度为24位,主机标识的长度为8位,C类网络地址数量较多,有209万余个网络。适用于小规模的局域网络,每个网络最多只能包含254台计算机。
- C类IP地址范围192.0.0.0-223.255.255.255
- D类地址
- D类IP地址在历史上被叫做多播地址(multicast address),即组播地址。在以太网中,多播地址命名了一组应该在这个网络中应用接收到一个分组的站点。
- 多播地址的最高位必须是“1110”,范围从224.0.0.0到239.255.255.255。
- 私网(局域网)
- 如,192.168.xxxx.xxxx,专门给组织内部使用的网络
案例:InetAddress类的使用
public class TestInetAddress {
public static void main(String[] args) throws Exception {
//查询本机地址
InetAddress inetAddress=InetAddress.getByName("127.0.0.1");
System.out.println(inetAddress);
InetAddress inetAddress1=InetAddress.getByName("localhost");
System.out.println(inetAddress1);
InetAddress inetAddress2=InetAddress.getLocalHost();
System.out.println(inetAddress2);
//查询网络IP地址
InetAddress inetAddress3=InetAddress.getByName("www.baidu.com");
System.out.println(inetAddress3);
//常用方法
// System.out.println(inetAddress3.getAddress());
System.out.println(inetAddress3.getCanonicalHostName());//获取规范域名
System.out.println(inetAddress3.getHostAddress());//获取ip
System.out.println(inetAddress3.getHostName());//获取域名
}
}案例:SocketAddress类的使用
public class TestSocketAddress {
public static void main(String[] args) {
InetSocketAddress socketAddress=new InetSocketAddress("127.0.0.1",8080);
InetSocketAddress socketAddress2=new InetSocketAddress("localhost",8080);
System.out.println(socketAddress);
System.out.println(socketAddress2);
System.out.println(socketAddress.getAddress());
System.out.println(socketAddress.getHostName());
System.out.println(socketAddress.getPort());
}
}端口
端口: 表示计算机上的一个程序的进程,不同的进程有不同的端口号,0~65535,单个协议下的端口不能冲突
- 公用端口:0~1023
- HTTP:80
- HTTPS:443
- Telent:23
- FTP:21
- 程序注册端口:1024~49151,分配给用户或者程序
- Tomcat:8080
- MySQL:3306
- Oracle:1521
- 动态,私有端口:49152~65535,尽量不要使用这些端口
- dos命名
- netstat -ano 查看所有端口
- netstat -ano|findstr "5900" 查看指定端口
- tasklist|findstr "8696" 查看指定端口的进程
通信协议
TCP:用户传输协议
- 特点:
- 通信时需要建立连接,稳定
- 客户端,服务端架构
- 传输完成,释放连接,效率低
- 三次握手建立连接,四次挥手断开连接
三次握手建立连接(计网的基础知识,在这里就不细讲了)
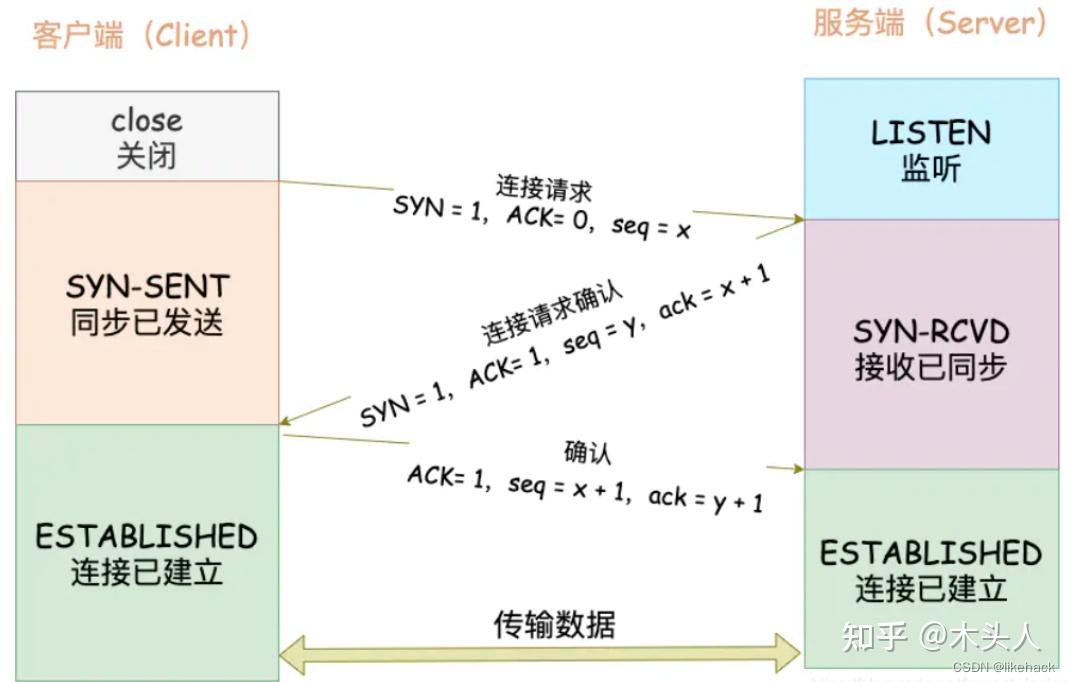
在这里插入图片描述
四次挥手断开连接
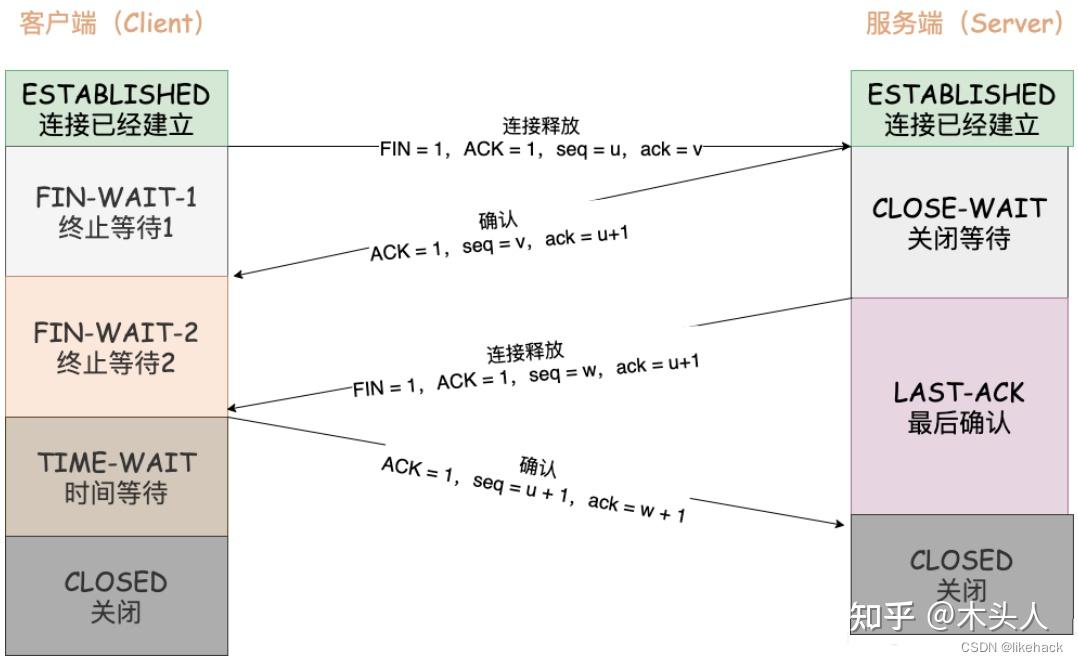
在这里插入图片描述
TCP实现通信
- 客户端 1,获取服务器地址 2,连接服务器Socket 3,发送消息
- 服务器 1,建立服务的端口 ServerSocket 2,等待用户的的连接 accept 3,接收用户的消息
运行时,要先打开服务器端,再打开客户端,这样客户端才能连接上服务器。结果在服务器端运行界面查看。
客户端
public class TcpClientDemo1 {
public static void main(String[] args) {
Socket socket=null;
OutputStream os=null;
try {
//1,获取服务器地址
InetAddress serverIP=InetAddress.getByName("127.0.0.1");
int port=9999;
//2,创建一个socket连接
socket=new Socket(serverIP,port);
//3,发送消息,IO流
os=socket.getOutputStream();
os.write("你好,欢迎学习java".getBytes());
} catch (Exception e) {
e.printStackTrace();
}finally {
if(socket!=null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(os!=null){
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}服务器端
public class TcpServerDemo1 {
public static void main(String[] args) {
ServerSocket serverSocket=null;
Socket socket=null;
InputStream is=null;
ByteArrayOutputStream baos=null;
try {
//1,建立服务器地址
serverSocket = new ServerSocket(9999);
//2,等待客户端连接
socket = serverSocket.accept();
//3,读取客户端消息
is=socket.getInputStream();
//管道流
baos=new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int len;
while ((len=is.read(buffer))!=-1){
baos.write(buffer,0,len);
}
System.out.println(baos);
} catch (Exception e) {
e.printStackTrace();
}finally {
//关闭资源
if(baos!=null){
try {
baos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(is!=null){
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(socket!=null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(serverSocket!=null){
try {
serverSocket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}TCP实现上传文件
客户端
public class TcpClientDemo2 {
public static void main(String[] args) throws Exception{
//1,创建一个socket连接
Socket socket=new Socket(InetAddress.getByName("127.0.0.1"),9000);
//2,创建一个输出流
OutputStream os=socket.getOutputStream();
//3,读取文件
FileInputStream fis=new FileInputStream(new File("2.jpg"));//这里的文件选择本地的一个文件即可
//4,写出文件
byte[] buffer=new byte[1024];
int len;
while ((len=fis.read(buffer))!=-1){
os.write(buffer,0,len);
}
//通知服务器,信息发送完毕
socket.shutdownOutput();
//检测服务器接收完毕,才能断开连接
InputStream is=socket.getInputStream();
ByteArrayOutputStream baos=new ByteArrayOutputStream();
byte[] buffer2=new byte[1024];
int len2;
while ((len2=is.read(buffer2))!=-1){
baos.write(buffer2,0,len2);
}
System.out.println(baos.toString());
//5,关闭资源
fis.close();
os.close();
socket.close();
}
}服务器端
public class TcpServerDemo2 {
public static void main(String[] args) throws Exception{
//1,创建服务器地址
ServerSocket serverSocket=new ServerSocket(9000);
//2,监听客户端连接
Socket socket=serverSocket.accept();
//3,获取输入流
InputStream is=socket.getInputStream();
//4,文件输出
FileOutputStream fos=new FileOutputStream(new File("receive.jpg"));
byte[] buffer=new byte[1024];
int len;
while ((len=is.read(buffer))!=-1){
fos.write(buffer,0,len);
}
//通知客户端接收完毕
OutputStream os=socket.getOutputStream();
os.write("接收完毕,可以断开连接".getBytes());
//5,关闭资源
fos.close();
is.close();
socket.close();
}
}UDP:用户数据报协议
- 特点
- 通信时不需要建立连接,不稳定
- 客户端,服务端架构:客户端和服务端没有明显界限
使用UDP实现通信
单次通信,发送固定内容
客户端
public class UdpClientDemo1 {
public static void main(String[] args) throws Exception {
//1,建立一个Socket
DatagramSocket socket=new DatagramSocket();
//2,创建数据包
String msg="客户端尝试连接服务器...服务器连接成功!";
//3,发送包
//发送的对象
InetAddress localhost=InetAddress.getByName("localhost");
int port=9090;
//发送的数据,数据的长度始,末,发送的对象,使用的端口
DatagramPacket packet=new DatagramPacket(msg.getBytes(),0,msg.getBytes().length,localhost,port);
//发送
socket.send(packet);
//关闭资源
socket.close();
}
}服务器端
public class UdpServerDemo1 {
public static void main(String[] args) throws Exception{
//开放端口
DatagramSocket socket=new DatagramSocket(9090);
//接收数据包
byte[] buffer=new byte[1024];
DatagramPacket packet = new DatagramPacket(buffer, 0, buffer.length);
socket.receive(packet);
System.out.println(new String(packet.getData()));
System.out.println(packet.getAddress().getHostAddress());
//关闭连接
socket.close();
}
}多次通信,内容由键盘输入
发送端
public class UdpSenderDemo1 {
public static void main(String[] args) throws Exception{
//1,建立一个Socket
DatagramSocket socket=new DatagramSocket(8888);
//2,创建数据包
//读取控制台输入
BufferedReader reader=new BufferedReader(new InputStreamReader(System.in));
while (true){
String data=reader.readLine();
byte[] datas=data.getBytes();
DatagramPacket packet=new DatagramPacket(datas,0,datas.length,new InetSocketAddress("localhost",6666));
//3,发送数据
socket.send(packet);
if(data.equals("bye")){
break;
}
}
//4,资源关闭
socket.close();
}
}接收端
public class UdpReceiveDemo1 {
public static void main(String[] args) throws Exception{
DatagramSocket socket=new DatagramSocket(6666);
while (true){
//循环检测,准备接收数据包
byte[] content=new byte[1024];
DatagramPacket packet=new DatagramPacket(content,0,content.length);
socket.receive(packet);
//检测如果发送的信息时bye结束聊天
byte[] data=packet.getData();
String reData=new String(data,0,data.length);
System.out.println(reData);
if(reData.trim().equals("bye")){
break;
}
}
}
}使用UDP实现聊天功能
发送端
public class TalkSend implements Runnable{
DatagramSocket socket=null;
BufferedReader reader=null;
private int fromPort;
private String toIP;
private int toPort;
public TalkSend(int fromPort,String toIP,int toPort){
this.fromPort=fromPort;
this.toIP=toIP;
this.toPort=toPort;
try {
socket=new DatagramSocket(fromPort);
reader=new BufferedReader(new InputStreamReader(System.in));
} catch (Exception e) {
e.printStackTrace();
}
}
@Override
public void run() {
while (true){
try {
String data=reader.readLine();
byte[] datas=data.getBytes();
DatagramPacket packet=new DatagramPacket(datas,0,datas.length,new InetSocketAddress(this.toIP,this.toPort));
//3,发送数据
socket.send(packet);
if(data.equals("bye")){
break;
}
} catch (IOException e) {
e.printStackTrace();
}
}
socket.close();
}
}接收端
public class TalkReceive implements Runnable{
DatagramSocket socket=null;
private int port;
private String msgFrom;
public TalkReceive(int port,String msgFrom){
this.port=port;
this.msgFrom=msgFrom;
try {
socket=new DatagramSocket(port);
} catch (SocketException e) {
e.printStackTrace();
}
}
@Override
public void run() {
while (true){
try {
//循环检测,准备接收数据包
byte[] content=new byte[1024];
DatagramPacket packet=new DatagramPacket(content,0,content.length);
socket.receive(packet);
//检测如果发送的信息时bye结束聊天
byte[] data=packet.getData();
String reData=new String(data,0,data.length);
System.out.println(msgFrom+":"+reData);
if(reData.equals("bye")){
break;
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
}聊天主体:学生
public class TalkStudent {
public static void main(String[] args) {
//发送信息给老师
new Thread(new TalkSend(8888,"localhost",9999)).start();
//从老师那接收信息
new Thread(new TalkReceive(7777,"老师")).start();
}
}聊天主体:老师
public class TalkTeacher {
public static void main(String[] args) {
//发送信息给学生
new Thread(new TalkSend(5555,"localhost",7777)).start();
//从学生那接收信息
new Thread(new TalkReceive(9999,"学生")).start();
}
}URL:统一资源定位符,定位互联网上的某一个资源
案例:URL类的基础使用
public class URLDemo1 {
public static void main(String[] args) throws Exception{
URL url = new URL("http://localhost:8080/helloworld/index.jsp?username=mtr&password=123");
System.out.println(url.getProtocol());//协议
System.out.println(url.getHost());//主机ip
System.out.println(url.getPort());//端口
System.out.println(url.getPath());//路径
System.out.println(url.getFile());//全路径
System.out.println(url.getQuery());//参数
}
}案例:使用URL下载网络文件
public class URLDown {
public static void main(String[] args) throws Exception{
//1,下载地址
URL url=new URL("https://ts1.cn.mm.bing.net/th/id/R-C.16fb679c22de598423282b5ac10ab64e?rik=gTeAbvnTPMPOcg&riu=http%3a%2f%2fpic.zsucai.com%2ffiles%2f2013%2f0830%2fxiaguang5.jpg&ehk=e63SK9oScdkmxiDRTgyUerCjF7AIu8xglQbXxtyur5M%3d&risl=&pid=ImgRaw&r=0");
//2,连接到这个资源
HttpURLConnection connection=(HttpURLConnection) url.openConnection();
InputStream is=connection.getInputStream();
FileOutputStream fos=new FileOutputStream("4.jpg");
byte[] buffer=new byte[1024];
int len;
while ((len=is.read(buffer))!=-1){
fos.write(buffer,0,len);
}
//3,关闭资源
is.close();
fos.close();
connection.disconnect();//断开连接
}
}其他相关文章
- Java知识梳理:Java知识梳理
- Java知识梳理--内部类:Java知识梳理--内部类
- Java常用类--Object类:Java常用类--Object类
- Java常用类--包装类:Java常用类--包装类
- Java常用类--String类:java常用类--String类
- Java常用类--时间相关类:Java常用类--时间相关类
- Java常用类--BigDecimal类和System类:Java常用类--BigDecimal类和System类
- Java集合--Collection集合:Java集合--Collection集合
- Java集合--泛型集合:Java集合--泛型集合
- Java集合--Map集合:Java集合--Map集合
- Java进阶--多线程:Java进阶--多线程
- Java进阶--I/O流:Java进阶--I/O流
|
|